The opposite of a variable is a constant. Constants are values that never change. Because of their inflexibility, constants are used less often than variables in programming. Therefore when we need data which always change we need to use variables for example this expression X + Y. X and Y are variables. Normally we use variables when we know the data range only for example user input data, calculation and etc.
Below Table is to show what data type to declare and what range it will be. 1st Table is for Numeric (Numbers) and 2nd Table is for Non Numeric (Other than numbers).
Numeric Data Types
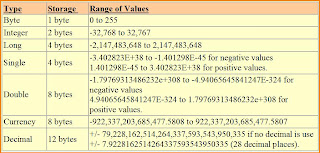
Non numeric Data Types
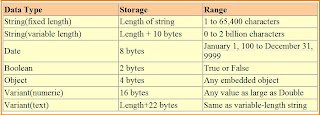
Every variable has a name, called the variable name, and a data type. A variable's data type indicates what sort of value the variable represents, such as whether it is an integer, a floating-point number, or a character. Variable name is not limit to X and Y only but you can use any name up to 255 characters if I’m not mistaken.
If you go to help in VBE the definition of variable as below:
Variable is a named storage location that can contain data that can be modified during program execution. Each variable has a name that uniquely identifies it within its scope. A data type can be specified or not.
Variable names must begin with an alphabetic character, must be unique within the same scope, can't be longer than 255 characters, and can't contain an embedded period or type-declaration character.
Actually you can use variable without specified data type but you have disadvantages:
- If you have very long code then it will be very difficult for you to do the editing because you do not know which variables belong to which function.
- Your program will be slower because it will consume your memory. If I’m not mistaken data type will be assume as variant (Highest range).
- Affect your program accuracy because sometime you only need only numbers but end result may be not numbers.
Therefore please consider to declare your data type for variables when you start writing.
Good Luck
Click here if you have any new project! Let us create for u for free.
Thanks